20 Key Coding Patterns That Will Guide You Through
Anyone can master these incredible algorithmic strategies and really excel in coding interviews by using roughly 20 of these coding issue patterns that are compiled here.
The idea behind these patterns is that one can use a pattern to solve dozens of issues once one becomes familiar with it.

Without further ado, let us enumerate each of these patterns:
1. Sliding Window
Usage: This algorithmic technique is used when we need to handle the input of the data in a specific window size.
DS involved: Array, String, HashTable

Sample Problems: Longest Substring with ‘K’ distinct characters, Fruits into baskets
2. Islands (Matrix Traversal)
Usage: This pattern describes all the efficient ways of traversing a matrix (or 2D array).
DS involved: Matrix, Queue

Sample Problem: Number of Islands, Flood Fill, Cycle in a matrix
3. Two Pointers
Usage: This technique uses two pointers to iterate input data. Generally, both pointers move in the opposite direction at a constant interval.
DS involved: Array, String, LinkedLists

Sample Problems: Squaring a Sorted Array, Dutch National Flag Problem, Minimum Window Sort
4. Fast & Slow Pointers
Usage: Also known as Hare & Tortoise algorithm. This technique uses two pointers that traverse the input data at different speeds.
DS involved: Array, String, LinkedList

Sample Problems: Middle of the LinkedList, Happy Number, Cycle in a Circular Array
5. Merge Intervals
Usage: This technique is used to deal with overlapping intervals.
DS involved: Array, Heap

Sample Problems: Conflicting Appointments, Minimum Meeting Rooms
6. Cyclic Sort
Usage: Use this technique to solve array problems where the input data lies within a fixed range.
DS involved: Array

Sample Problems: Find All Missing Numbers, Find All Duplicate Numbers, Find the First K mIssing Positive Numbers
7. In-place Reversal of a LinkedList
Usage: This technique provides an efficient way to reverse the links between a set of nodes of a LinkedList. Often, the constraint is that we need to do this in place, i.e., using the existing node objects and without using extra memory.
DS involved: LinkedList

Sample Problems: Reverse every K-element Sub-list
8. Tree Breadth-First Search
Usage: This technique is used to solve problems involving traversing trees or graphs in a breadth-first search manner.
DS involved: Trees, Graph, Matrix, Queue
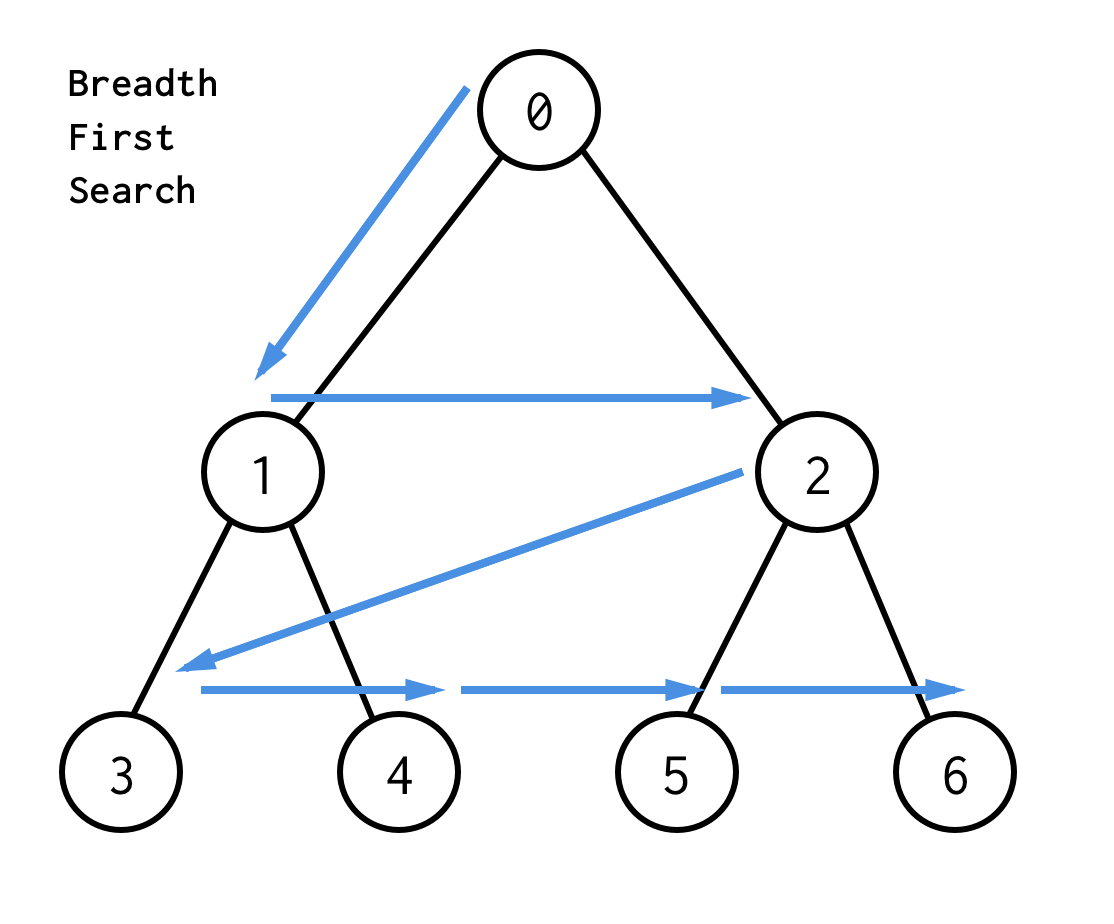
Sample Problems: Binary Tree Level Order Traversal, Minimum Depth of a Binary Tree, Connect Level Order Siblings
9. Tree Depth First Search
Usage: This technique is used to solve problems involving how traversing trees or graphs in a depth-first searching manner.
DS involved: Trees, Graphs, Matrix

Sample Problems: Path With Given Sequence, Count Paths for a sum
10. Two Heaps
Usage: In many problems, we are given a set of elements that can be divided into two parts. We are interested in knowing the smallest element in one part and the biggest element in the other part. As the name suggests, this technique uses a Min-Heap to find the smallest element and a Max-Heap to find the biggest element.
DS involved: Heap, Array
Sample Problems; Find the median of a number system, Next Interval

11. Subsets
Usage: Use this technique when the problem asks to deal with permutations or combinations of a set of elements.
DS Involved: Queue, Array, String
Sample Problems: String permutations by changing case, Unique Generalized Abbreviations
12. Modified Binary Search
Usage: Use this technique to search a sorted set of elements efficiently.
DS involved: Array
Sample Problems: Ceiling of a Number, Bitonic Array Maximum
13. Bitwise XOR
Usage: This technique uses the XOR operator to manipulate bits to solve problems.
DS involved: Array, Bits
Sample Problems: Two Single Numbers, Flip and Invert an Image
14. Top ‘K’ Elements
Usage: This technique is used to find the top/smallest/frequently occurring ’K’ elements in a set.
DS involved: Array, Heap, Queue
Sample Problems: ‘K’ Closest Points to the Origin, Maximum Distinct Elements
15. K-way Merge
Usage: This technique helps us to solve problems that involve a list of sorted arrays.
DS involved: Array, Queue, Heap
Sample Problems: Kth Smallest Number in M sorted Lists, Kth Smallest Numbers in a Sorted Matrix
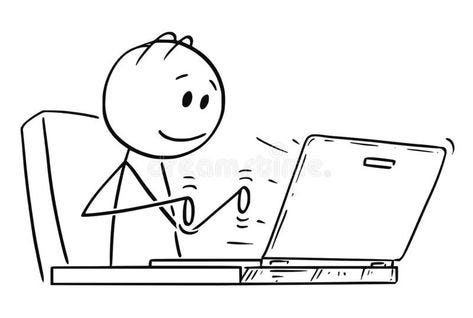
16. Topological Sort
Usage: This technique is used to find a linear ordering of elements that have dependencies on each other.
DS involved: Array, HashTable, Queue, Graph

Sample Problems: Tasks Scheduling, Alien Dictionary
17. 0/1 Knapsack
Usage: This technique is used to solve optimization problems. Use this technique to select elements that give maximum profit from a given set with limitations on capacity and that each element can only be picked once.
DS involved: Array, HashTable
Sample Problems: Equal Subset Sum Partition, Minimum Subset Sum Difference
18. Fibonacci Numbers
Usage: Use this technique to solve problems that follow the Fibonacci numbers sequence, i.e., every subsequent number is calculated from the last few numbers.
DS involved: Array, HashTable

Sample Problems: Staircase, HouseTheif
19. Palindromic Subsequence
Usage: This technique is used to solve optimization problems related to palindromic sequences or strings.
DS involved: Array, HashTable
Sample Problems: Longest Palindromic Subsequence, Minimum Deletions in a String to make it a Palindrome

20. Longest Common Substring
Usage: Use this string technique to find the optimal part of a string/sequence or set of strings/ sequences.
DS involved: Array, HashTable
Sample Problems: Maximum Sum Increasing Subsequence, Edit Distance
DSAP 20 (Total 75 – 85 problems)
//…………………………………………………………………………..
Topic 1 –> Binary Search
Problem 1 –> Simple Binary Search https://leetcode.com/problems/binary-search/
Problem 2 –> Search in Rotated Sorted Array (Array is twisted) https://leetcode.com/problems/search-in-rotated-sorted-array/
Problem 3 –> Peak Element (Binary Search on Answer) https://leetcode.com/problems/find-peak-element/
Problem 4 –> Allocate Book Pages (Array is not sorted) https://practice.geeksforgeeks.org/problems/allocate-minimum-number-of-pages0937/1
Problem 5 → Longest Increasing Subsequence (Also included in DP)
https://leetcode.com/problems/longest-increasing-subsequence
//…………………………………………………………………………..
Topic 2 –> Hashing (HashMap and HashSet)
Problem 1 –> Longest Subarray with given sum https://practice.geeksforgeeks.org/problems/longest-sub-array-with-sum-k0809/1
Problem 2 –> Count Subarray With Given Xor https://www.interviewbit.com/problems/subarray-with-given-xor/
Problem 3 → Longest Consecutive Sequence
https://leetcode.com/problems/longest-consecutive-sequence
//…………………………………………………………………………..
Topic 3–> PriorityQueue
Problem 1 → Kth Largest Element in an array
https://leetcode.com/problems/kth-largest-element-in-an-array
Problem 2 –> K closest point to origin https://leetcode.com/problems/k-closest-points-to-origin/
Problem 3 –> Find Median of a number stream (Two Heap Problem) https://leetcode.com/problems/find-median-from-data-stream/
//…………………………………………………………………………..
Topic 4 –> Two Pointers
Problem 1 –> 3 sum problem (Medium) https://leetcode.com/problems/3sum/
Problem 2 –> Container with most water (Medium) https://leetcode.com/problems/container-with-most-water/
Problem 3 –>Longest Palindromic Substring (Medium) https://leetcode.com/problems/longest-palindromic-substring/
Problem 4 –> Trapping Rainwater (Hard) https://leetcode.com/problems/trapping-rain-water/
//…………………………………………………………………………..
Topic 5 –> Sliding Window
Problem 1 –> Sliding window maximum (Hard Fixed size sliding window) https://leetcode.com/problems/sliding-window-maximum/description/
Problem 2 –> String anagrams (Medium size sliding window) https://leetcode.com/problems/find-all-anagrams-in-a-string/
Problem 3 –> Longest substring without Repeating characters. (Medium Variable size window) https://leetcode.com/problems/longest-substring-without-repeating-characters/
Problem 4 –> Fruit into Basket (Medium variable size sliding window) https://leetcode.com/problems/fruit-into-baskets/
Practice Problems → Leetcode 713 , Leetcode 2962 (These two problems are of counting the subarrays)
//…………………………………………………………………………..
Topic 6 –> Cyclic Sort
Problem 1 –> Missing Number https://leetcode.com/problems/missing-number/
Problem 2 –> First Positive Number https://leetcode.com/problems/first-missing-positive/
Practice problems → Leetcode 448, 287, 442, 645
//…………………………………………………………………………..
Topic 7 –> Merge Intervals
Problem 1 –> Merge Intervals https://www.interviewbit.com/problems/merge-intervals/
Problem 2 –> Merge Overlapping Intervals https://www.interviewbit.com/problems/merge-overlapping-intervals/
Practice Problem → Leetcode 452
//…………………………………………………………………………………
Topic 8 – -> Greedy Algorithm
Problem 1 –> Minimum Platform https://practice.geeksforgeeks.org/problems/minimum-platforms-1587115620/1?utm_source=youtube&utm_medium=collab_anujbhaiya_description&utm_campaign=minimum-platforms
Problem 2 –> Candy Distribution Problem https://leetcode.com/problems/candy/
Practice problem → leetcode 621
//…………………………………………………………………………..
Topic 9 –> Recursion and Backtracking
Problem 1 –> Josephus Problem https://leetcode.com/problems/find-the-winner-of-the-circular-game/description/
Problem 2 –> Unique Path https://leetcode.com/problems/unique-paths/
Problem 3 –> N Queen Problem https://leetcode.com/problems/n-queens/
Problem 4 –> Subsets (power Set) https://leetcode.com/problems/subsets/
Problem 5 –> Combination 1 https://leetcode.com/problems/combination-sum/
Problem 6 –> Combination 2 https://leetcode.com/problems/combination-sum-ii/
Problem 7 –> Permutation 1 https://leetcode.com/problems/permutations/
Problem 8 –> Permutation 2 https://leetcode.com/problems/permutations-ii/description/
//……………………………………………………………………………….
Topic 10 –> Fast and slow pointer (LinkedList)
Problem 1 –> Linked List Cycle https://leetcode.com/problems/linked-list-cycle/
Problem 2 –> Palindrome Linked List https://leetcode.com/problems/palindrome-linked-list/
//…………………………………………………………………………………….
Topic 11 –> LinkedList (Some Important Problems)
Problem 1 –> Reverse a LinkedList (easy) https://leetcode.com/problems/reverse-linked-list/
Problem 2 –> Reverse Nodes in k-Group (hard) https://leetcode.com/problems/reverse-nodes-in-k-group/
Problem 3 –> Merge K Sorted list (hard) https://leetcode.com/problems/merge-k-sorted-lists/
//……………………………………………………………………………………………………
Topic 12 –> Stack
Problem 1 –> Next Greater Element https://leetcode.com/problems/next-greater-element-i/
Problem 2 –> Largest Area Histogram https://leetcode.com/problems/largest-rectangle-in-histogram/
Problem 3 → Minimum Stack
https://leetcode.com/problems/min-stack
//……………………………………………………………………………………………………
Topic 13 –> Dynamic Programming
Problem 1 –> 0/1 KnapSack https://practice.geeksforgeeks.org/problems/0-1-knapsack-problem0945/1
Problem 2 –> Coin Change 1 https://leetcode.com/problems/coin-change/
Problem 3 –> Coin Change 2 https://leetcode.com/problems/coin-change-ii/
Problem 4 –> Longest Common Subsequence https://leetcode.com/problems/longest-common-subsequence/
Applications Problem 4.1 —> Longest Palindromic Subsequence https://leetcode.com/problems/longest-palindromic-subsequence/
Problem 4.2 —> Minimum Insertion Steps to make a String Palindrome https://leetcode.com/problems/minimum-insertion-steps-to-make-a-string-palindrome/
Problem 5 –> Matrix Chain Multiplication https://practice.geeksforgeeks.org/problems/matrix-chain-multiplication0303/1
Application Problem 5.1 –> Burst Balloon https://leetcode.com/problems/burst-balloons/
Problem 6 –> Longest Increasing Subsequence https://leetcode.com/problems/longest-increasing-subsequence/
Problem 7 –> Edit Distance https://leetcode.com/problems/edit-distance/
//……………………………………………………………………………………..………
Topic 14 –> Some Problems on Binary Tree
Problem 1 –> DFS (Inorder, PostOrder and PreOrder) https://leetcode.com/problems/binary-tree-inorder-traversal/
Problem 2 –> Level Order Traversal https://leetcode.com/problems/binary-tree-level-order-traversal/
Problem 3 –> Vertical order Traversal https://leetcode.com/problems/vertical-order-traversal-of-a-binary-tree/
Problem 4 –> Height of a Binary Tree https://practice.geeksforgeeks.org/problems/height-of-binary-tree/1
Problem 5 –> LCA in Binary Tree https://practice.geeksforgeeks.org/problems/lowest-common-ancestor-in-a-binary-tree/1
Problem 6 –> Construct Binary Tree from Preorder and Inorder Traversal https://leetcode.com/problems/construct-binary-tree-from-preorder-and-inorder-traversal/
//…………………………………………………………………………………………
Topic –> 15 (Binary Search Tree)
Problem 1 –> LCA in Binary Search Tree https://practice.geeksforgeeks.org/problems/lowest-common-ancestor-in-a-bst/1
Problem 2 –> isBST https://practice.geeksforgeeks.org/problems/check-for-bst/1
Problem 3 –> Largest BST https://practice.geeksforgeeks.org/problems/largest-bst/1
//…………………………….………………………………………………………………..
Topic 16 –> Dp on trees
Problem 1 –> Diameter of a tree https://practice.geeksforgeeks.org/problems/diameter-of-binary-tree/1
Problem 2 —> Maximum Path Sum https://leetcode.com/problems/binary-tree-maximum-path-sum/
Problem 3 —> Longest Zig-Zag path in a Binary Tree https://leetcode.com/problems/longest-zigzag-path-in-a-binary-tree/
//……………………………………………………………………………………………
Topic 17 –> Some Important Graph Problem
Problem 1 –> DFS https://practice.geeksforgeeks.org/problems/depth-first-traversal-for-a-graph/1
Problem 2 –> BFS https://practice.geeksforgeeks.org/problems/bfs-traversal-of-graph/1
Problem 3 –> Cycle in undirected Graph https://practice.geeksforgeeks.org/problems/detect-cycle-in-an-undirected-graph/1
Problem 4 –> Cycle in Directed Graph (Course Schedule) https://leetcode.com/problems/course-schedule/description/
Problem 5 –> topological sort https://practice.geeksforgeeks.org/problems/topological-sort/1
Problem 6 –> Rotten Oranges (Multi Source BFS) https://leetcode.com/problems/rotting-oranges/
Problem 7 –> Union Find (isBipartite) https://practice.geeksforgeeks.org/problems/bipartite-graph/1
//……………………………………………………………………………………………………
Topic 18 –> Trie
Problem 1 –> Insert and Delete in a trie https://practice.geeksforgeeks.org/problems/trie-insert-and-search0651/1
Problem 2 –> Xor Problem https://practice.geeksforgeeks.org/problems/maximum-xor-of-two-numbers-in-an-array/0?utm_source=youtube&utm_medium=collab_anujbhaiya_description&utm_campaign=two-numbers-in-an-array
//……………………………………………………………………………………………………
Topic 19 –> XOR related Problems
Problem 1 –> Single Number https://leetcode.com/problems/single-number/
Problem 2 –> Single Number III https://leetcode.com/problems/single-number-iii/
Problem 3 –> Single Number II https://leetcode.com/problems/single-number-ii/
// …………………………………………………………………………………………..
Topic 20 –> Must Know Algorithms
Problem 1 –> Kadane’s Algorithm https://www.geeksforgeeks.org/largest-sum-contiguous-subarray/
Problem 2 –> Moore Algo https://leetcode.com/problems/majority-element/
Problem 3 –> Dutch National Flag https://leetcode.com/problems/sort-colors/
Problem 4 –> Celebrity Problem https://practice.geeksforgeeks.org/problems/the-celebrity-problem/1?utm_source=gfg&utm_medium=article&utm_campaign=bottom_sticky_on_article
Problem 5 –> Merge Sort https://practice.geeksforgeeks.org/problems/merge-sort/1
Problem 6 –> Quick Sort https://practice.geeksforgeeks.org/problems/quick-sort/1
Total Problems –> 77